- Published on
- 🍵 4 min read
Building a Multi-Agent Team for Job Search with PhiData
- Authors
- Name
- Emin Vergil
- @eminvergil
Overview
- Introduction
- What We’re Building
- Agents
- HackerNews Researcher Agent
- Web Searcher Agent
- Job Search Team
- Example Response
- Full Code
Introduction
In my previous post, I covered how to build a simple agent using the Phidata framework. This time, we’ll take it a step further and create a multi-agent team using Phidata.
The job search agent in this tutorial is just for demonstration purposes. It’s not intended for real-world job applications, so use it cautiously.
What We’re Building
In this tutorial, we’ll create a job search agent that collaborates with two specialized agents:
- HackerNews Researcher Agent – Finds top stories and discussions about tech hiring and relevant companies on Hacker News.
- Web Search Agent – Searches the web (via DuckDuckGo) for job listings that match the candidate’s profile.
We’ll be using Gemini as our AI model. You can get an API key from Google AI Studio.
We'll also give the agent candidate details to help find the best job matches.
Agents
HackerNews Researcher Agent
hn_researcher = Agent(
name="HN Researcher",
role="Finds top stories and discussions related to tech hiring and relevant companies on Hacker News.",
tools=[HackerNews()],
model=gemini_model
)
Web Searcher Agent
web_searcher = Agent(
name="Web Searcher",
role="Searches the web (DuckDuckGo) for job listings matching the candidate’s profile.",
tools=[DuckDuckGo()],
model=gemini_model,
add_datetime_to_instructions=True,
)
Job Search Team
jb_team = Agent(
name="Job Team",
team=[hn_researcher, web_searcher],
instructions=[
"Find remote job postings for the candidate.",
"HN Researcher: Provide context, recent trends, and company insights.",
"Web Searcher: Find job postings and URLs that match the candidate's skills and location.",
"Present the results as a simple list of job links with company names."
],
show_tool_calls=True,
model=gemini_model,
markdown=True,
)
Example Response
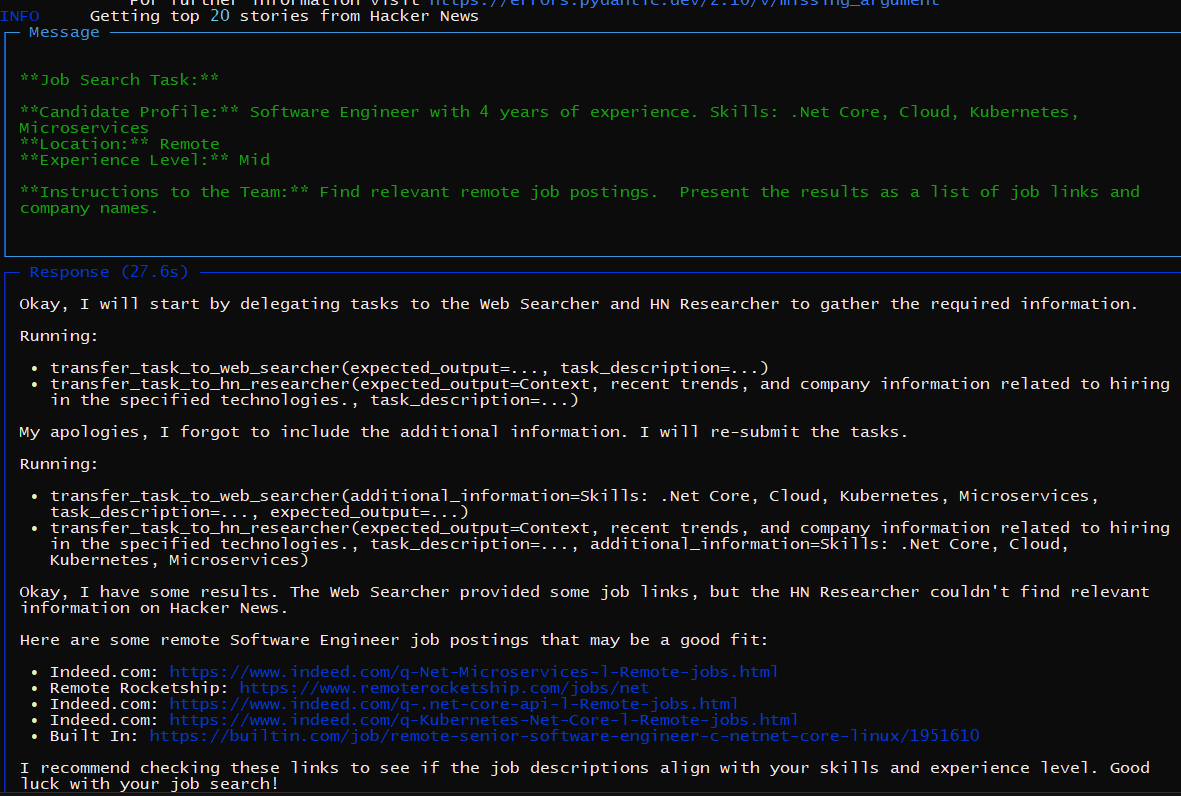
Full Code
from phi.agent import Agent
from phi.tools.hackernews import HackerNews
from phi.tools.duckduckgo import DuckDuckGo
from phi.tools.newspaper4k import Newspaper4k
from phi.model.google import Gemini
gemini_model = Gemini(api_key="")
hn_researcher = Agent(
name="HN Researcher",
role="Finds top stories and discussions related to tech hiring and relevant companies on Hacker News.",
tools=[HackerNews()],
model=gemini_model
)
web_searcher = Agent(
name="Web Searcher",
role="Searches the web (DuckDuckGo) for job listings matching the candidate’s profile.",
tools=[DuckDuckGo()],
model=gemini_model,
add_datetime_to_instructions=True,
)
jb_team = Agent(
name="Job Team",
team=[hn_researcher, web_searcher],
instructions=[
"Find remote job postings for the candidate.",
"HN Researcher: Provide context, recent trends, and company insights.",
"Web Searcher: Find job postings and URLs that match the candidate's skills and location.",
"Present the results as a simple list of job links with company names."
],
show_tool_calls=True,
model=gemini_model,
markdown=True,
)
# Candidate details (to be filled in)
candidate_info = ""
location = ""
experience_level = ""
# Run the agent team
jb_team.print_response(
f"""
**Job Search Task:**
**Candidate Profile:** {candidate_info}
**Location:** {location}
**Experience Level:** {experience_level}
**Instructions to the Team:** Find relevant remote job postings and present the results as a list of job links and company names.
""",
stream=True
)
You can also check similar posts about phidata: