- Published on
- 🍵 5 min read
Integrating Elasticsearch with .NET Aspire
- Authors
- Name
- Emin Vergil
- @eminvergil
Overview
- Integrating Elasticsearch with .NET Aspire
- Step 1: Install the Elasticsearch Hosting Package
- Step 2: Add Elasticsearch to Your App Host
- Step 3: Install the Elasticsearch Client Package
- Step 4: Set Up the Client in Your Services
- Step 5: Use the ElasticsearchClient in Your Services
- Testing Your Setup
- Quick Example: Indexing and Searching
Integrating Elasticsearch with .NET Aspire
.NET Aspire has a lot of integrations like Redis, MongoDB, PostgreSQL, RabbitMQ, and more. In this blog post, we’ll focus on Elasticsearch integration.
Elasticsearch is great for searching and indexing data quickly. It’s built on top of Lucene and provides a powerful way to search through your data.
Step 1: Install the Elasticsearch Hosting Package
dotnet add package Aspire.Hosting.Elasticsearch --version 9.1.0-preview.1.25121.10
Step 2: Add Elasticsearch to Your App Host
var builder = DistributedApplication.CreateBuilder(args);
var elasticsearch = builder.AddElasticsearch("elasticsearch")
.WithDataVolume();
builder.AddProject<Projects.Content_Api>("content-api")
.WithReference(elasticsearch);
builder.Build().Run();
This sets up an Elasticsearch instance called "elasticsearch" and links it to your "content-api" project. All .NET Aspire container resources can utilize volume mounts, and some provide convenient APIs for adding named volumes derived from resources. The WithDataVolume()
keeps your data safe even after restarts.
Step 3: Install the Elasticsearch Client Package
dotnet add package Aspire.Elastic.Clients.Elasticsearch --version 9.1.0-preview.1.25121.10
Step 4: Set Up the Client in Your Services
builder.AddElasticsearchClient("elasticsearch", configureClientSettings: clientSettings => clientSettings.DefaultIndex("contents"));
We’ve set a default index called contents
. However, you can also define custom indexes by using the Index("index_name")
method.
Step 5: Use the ElasticsearchClient in Your Services
You can retrieve the ElasticsearchClient
instance using dependency injection
public class ExampleService(ElasticsearchClient client)
{
// Use client...
}
Testing Your Setup
Once your AppHost is running, open the .NET Aspire Dashboard to verify your Elasticsearch resource. Look at the environment variables to find details about your Elasticsearch instance. You can also check the version and build details by checking resource endpoint.
Use the username elastic
and grab the password from the ELASTIC_PASSWORD
variable in the Elasticsearch resource's environment variables.
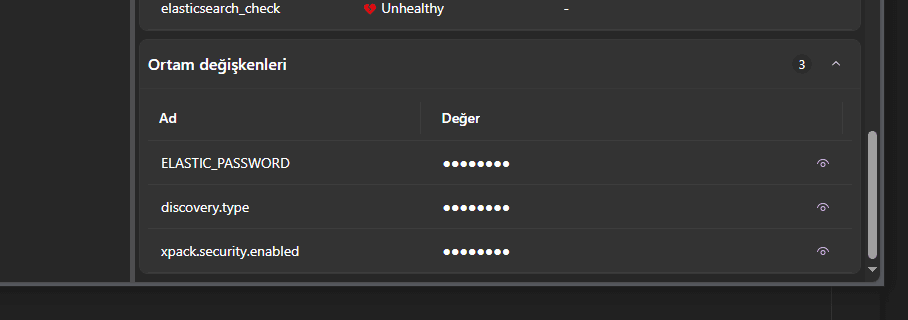
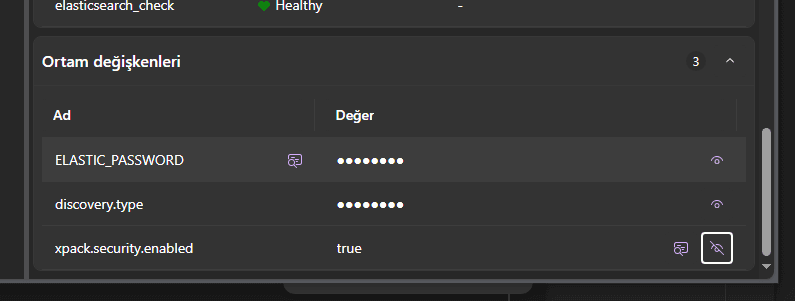
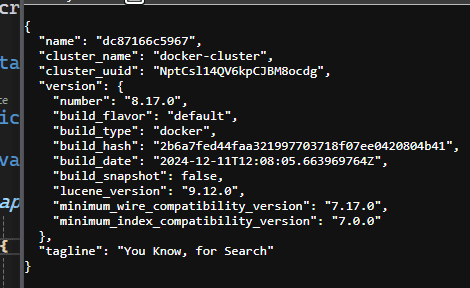
Disabling Security for Local Testing
Elasticsearch has security on by default. If you want to disable it locally, you can set the xpack.security.enabled
environment variable to false
.
var elasticsearch = builder.AddElasticsearch("elasticsearch")
.WithEnvironment("xpack.security.enabled", "false")
.WithDataVolume();
Note: This is only for testing purposes. Do not disable security in production.
Quick Example: Indexing and Searching
Step 1: Create a simple data model
public record ContentModel(string Name, string Description);
Step 2: Add an Insert Endpoint
app.MapPost("/insert", async Task<Results<Ok<string>, ProblemHttpResult>> (ContentModel item, ElasticsearchClient client) =>
{
var response = await client.IndexAsync(item);
return response.IsValidResponse
? TypedResults.Ok("Document inserted successfully.")
: TypedResults.Problem(response.DebugInformation);
});
Step 3: Add a Search Endpoint
app.MapPost("/search", async Task<Results<Ok<IReadOnlyCollection<ContentModel>>, ProblemHttpResult>> (string text, ElasticsearchClient client) =>
{
var response = await client.SearchAsync<ContentModel>(s => s
.Query(q => q
.Wildcard(w => w
.Field(i => i.Name)
.Value($"*{text}*")
)
)
);
return response.IsValidResponse
? TypedResults.Ok(response.Documents)
: TypedResults.Problem(response.DebugInformation);
});
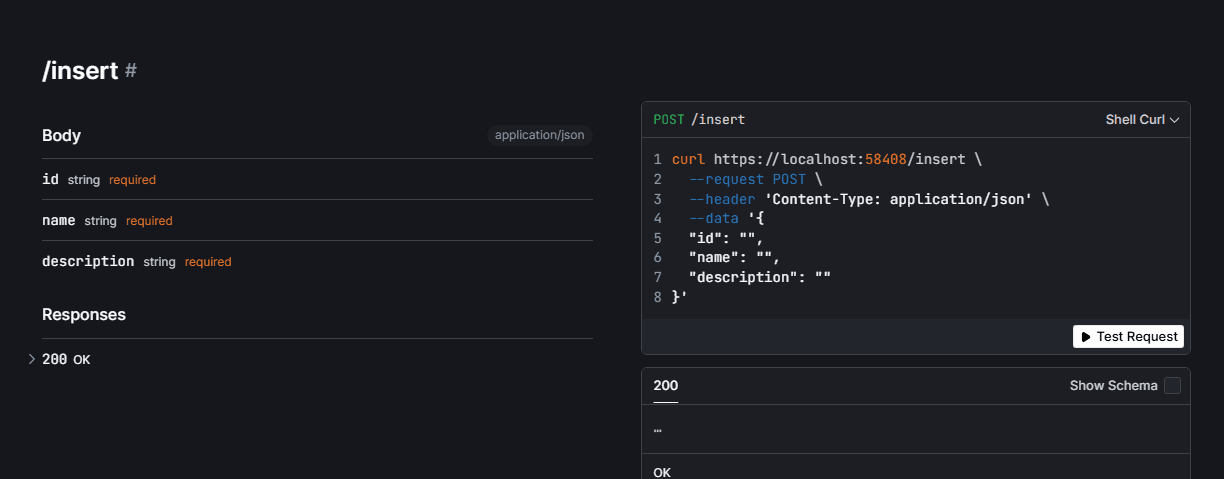
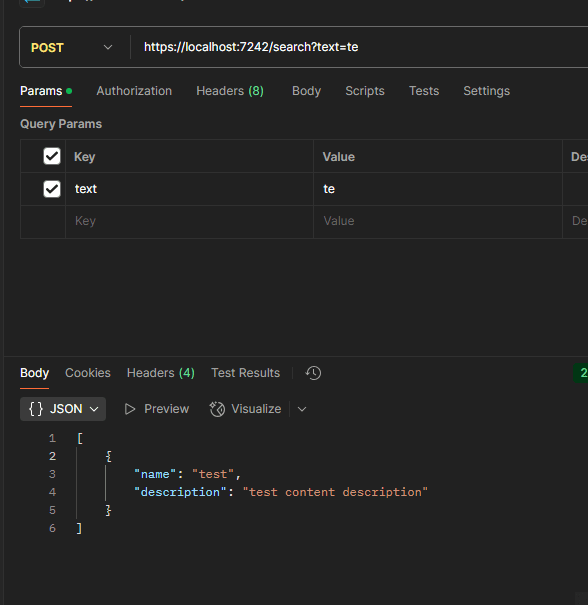
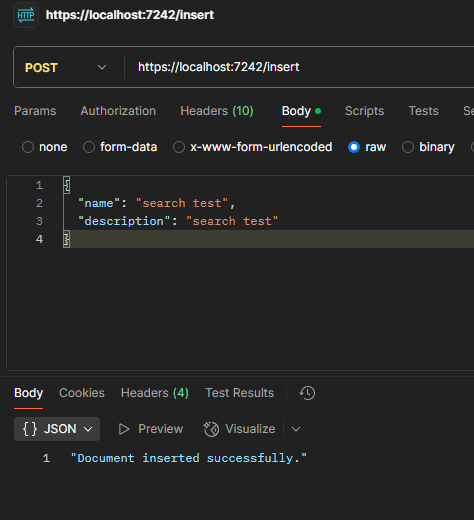
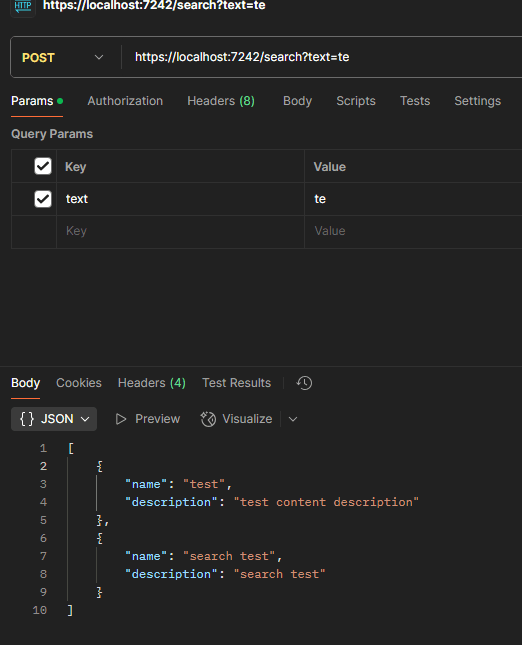
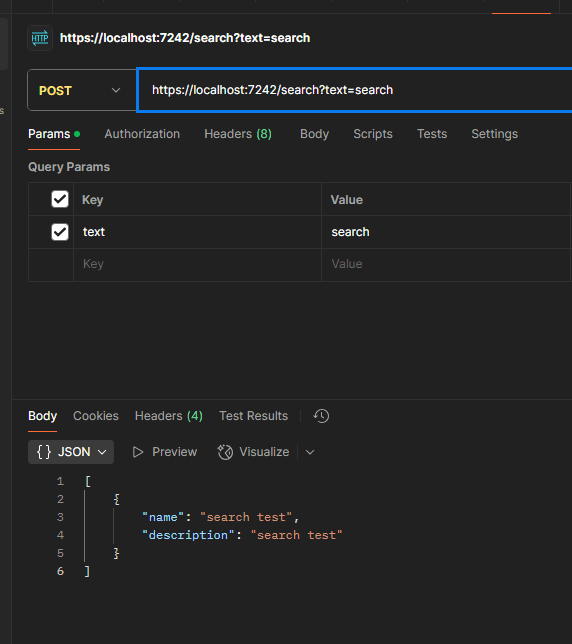
Source code for the example can be found here
You can also check similar posts about .NET Aspire:
- Deploying .NET Aspire Microservices to Kubernetes with Aspir8 CLI and Helm
- Aspire Kubernetes Deployment
- Redis Pub/Sub with Aspire and .NET
- Aspire Azure Emulators